- Science, Tech, Math ›
- Computer Science ›
- Java Programming ›

Compound-Assignment Operators
- Java Programming
- PHP Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- M.A., Advanced Information Systems, University of Glasgow
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound-Assignment Operators in Java
Java supports 11 compound-assignment operators:
Example Usage
To assign the result of an addition operation to a variable using the standard syntax:
But use a compound-assignment operator to effect the same outcome with the simpler syntax:
- Java Expressions Introduced
- Understanding the Concatenation of Strings in Java
- Conditional Operators
- Conditional Statements in Java
- Working With Arrays in Java
- Declaring Variables in Java
- If-Then and If-Then-Else Conditional Statements in Java
- How to Use a Constant in Java
- Common Java Runtime Errors
- What Is Java?
- What Is Java Overloading?
- Definition of a Java Method Signature
- What a Java Package Is In Programming
- Java: Inheritance, Superclass, and Subclass
- Understanding Java's Cannot Find Symbol Error Message
- Using Java Naming Conventions
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Compound assignment operators in Java
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java:
Implementation of all compound assignment operator
Rules for resolving the Compound assignment operators
At run time, the expression is evaluated in one of two ways.Depending upon the programming conditions:
- First, the left-hand operand is evaluated to produce a variable. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, the value of the left-hand operand is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the saved value of the left-hand variable and the value of the right-hand operand are used to perform the binary operation indicated by the compound assignment operator. If this operation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the result of the binary operation is converted to the type of the left-hand variable, subjected to value set conversion to the appropriate standard value set, and the result of the conversion is stored into the variable.
- First, the array reference sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the index sub-expression (of the left-hand operand array access expression) and the right-hand operand are not evaluated and no assignment occurs.
- Otherwise, the index sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, if the value of the array reference sub-expression is null, then no assignment occurs and a NullPointerException is thrown.
- Otherwise, the value of the array reference sub-expression indeed refers to an array. If the value of the index sub-expression is less than zero, or greater than or equal to the length of the array, then no assignment occurs and an ArrayIndexOutOfBoundsException is thrown.
- Otherwise, the value of the index sub-expression is used to select a component of the array referred to by the value of the array reference sub-expression. The value of this component is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
Examples : Resolving the statements with Compound assignment operators
We all know that whenever we are assigning a bigger value to a smaller data type variable then we have to perform explicit type casting to get the result without any compile-time error. If we did not perform explicit type-casting then we will get compile time error. But in the case of compound assignment operators internally type-casting will be performed automatically, even we are assigning a bigger value to a smaller data-type variable but there may be a chance of loss of data information. The programmer will not responsible to perform explicit type-casting. Let’s see the below example to find the difference between normal assignment operator and compound assignment operator. A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T) ((E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once.
For example, the following code is correct:
and results in x having the value 7 because it is equivalent to:
Because here 6.6 which is double is automatically converted to short type without explicit type-casting.
Refer: When is the Type-conversion required?
Explanation: In the above example, we are using normal assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) that’s results in compile time error. Here we have to do type-casting to get the result.
Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type-casting is automatically done by compile. Here we don’t have to do type-casting to get the result.
Reference: http://docs.oracle.com/javase/specs/jls/se7/html/jls-15.html#jls-15.26.2
Similar Reads
- Compound assignment operators in Java Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java: 1. += (compound addition 7 min read
- Java Assignment Operators with Examples Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they 7 min read
- Basic Operators in Java Java provides a rich operator environment. We can classify the basic operators in java in the following groups: Arithmetic OperatorsRelational OperatorsBitwise OperatorsAssignment OperatorsLogical Operators Let us now learn about each of these operators in detail. 1. Arithmetic Operators: Arithmetic 10 min read
- Double colon (::) operator in Java The double colon (::) operator, also known as method reference operator in Java, is used to call a method by referring to it with the help of its class directly. They behave exactly as the lambda expressions. The only difference it has from lambda expressions is that this uses direct reference to th 4 min read
- Bitwise Operators in Java Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they 6 min read
- Difference between Simple and Compound Assignment in Java Many programmers believe that the statement "x += i" is simply a shorthand for "x = x + i". This isn’t quite true. Both of these statements are assignment expressions. The second statement uses the simple assignment operator (=), whereas the first uses a compound assignment operator. The compound as 2 min read
- dot(.) Operator in Java The dot (.) operator is one of the most frequently used operators in Java. It is essential for accessing members of classes and objects, such as methods, fields, and inner classes. This article provides an in-depth look at the dot operator, its uses, and its importance in Java programming. Dot(.) Op 4 min read
- Addition and Concatenation Using + Operator in Java Till now in Java, we were playing with the integral part where we witnessed that the + operator behaves the same way as it was supposed to because the decimal system was getting added up deep down at binary level and the resultant binary number is thrown up at console in the generic decimal system. 2 min read
- & Operator in Java with Examples The & operator in Java has two definite functions: As a Relational Operator: & is used as a relational operator to check a conditional statement just like && operator. Both even give the same result, i.e. true if all conditions are true, false if any one condition is false. However, 2 min read
- Java Arithmetic Operators with Examples Operators constitute the basic building block to any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they 6 min read
- || operator in Java || is a type of Logical Operator and is read as "OR OR" or "Logical OR". This operator is used to perform "logical OR" operation, i.e. the function similar to OR gate in digital electronics. One thing to keep in mind is the second condition is not evaluated if the first one is true, i.e. it has a sh 1 min read
- && operator in Java with Examples && is a type of Logical Operator and is read as "AND AND" or "Logical AND". This operator is used to perform "logical AND" operation, i.e. the function similar to AND gate in digital electronics. One thing to keep in mind is the second condition is not evaluated if the first one is false, i. 1 min read
- Shift Operator in Java Operators in Java are used to performing operations on variables and values. Examples of operators: +, -, *, /, >>, <<. Types of operators: Arithmetic Operator,Shift Operator,Relational Operator,Bitwise Operator,Logical Operator,Ternary Operator andAssignment Operator. In this article, w 4 min read
- Interesting facts about Increment and Decrement operators in Java Increment operator is used to increment a value by 1. There are two varieties of increment operator: Post-Increment: Value is first used for computing the result and then incremented.Pre-Increment: Value is incremented first and then the result is computed.Example [GFGTABS] Java //INCREMENTAL OPERA 5 min read
- Java Operators Java operators are special symbols that perform operations on variables or values. They can be classified into several categories based on their functionality. These operators play a crucial role in performing arithmetic, logical, relational, and bitwise operations etc. Example: Here, we are using + 14 min read
- CompoundName compareTo() method in Java with Examples The compareTo() method of a javax.naming.CompoundName class is used to compare this CompoundName with the specified object passed as a parameter. It returns a negative integer, zero, or a positive integer as this CompoundName object is less than, equal to, or greater than the given Object as a param 2 min read
- Diamond operator for Anonymous Inner Class with Examples in Java Prerequisite: Anonymous Inner Class Diamond Operator: Diamond operator was introduced in Java 7 as a new feature.The main purpose of the diamond operator is to simplify the use of generics when creating an object. It avoids unchecked warnings in a program and makes the program more readable. The dia 2 min read
- How compare() method works in Java Prerequisite: Comparator Interface in Java, TreeSet in JavaThe compare() method in Java compares two class specific objects (x, y) given as parameters. It returns the value: 0: if (x==y)-1: if (x < y)1: if (x > y)Syntax: public int compare(Object obj1, Object obj2)where obj1 and obj2 are the t 3 min read
- Bitwise Right Shift Operators in Java In C/C++ there is only one right shift operator '>>' which should be used only for positive integers or unsigned integers. Use of the right shift operator for negative numbers is not recommended in C/C++, and when used for negative numbers, the output is compiler dependent. Unlike C++, Java su 2 min read
- Java-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators
- 8 contributors
expression assignment-operator expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
Assignment operators store a value in the object specified by the left operand. There are two kinds of assignment operations:
simple assignment , in which the value of the second operand is stored in the object specified by the first operand.
compound assignment , in which an arithmetic, shift, or bitwise operation is performed before storing the result.
All assignment operators in the following table except the = operator are compound assignment operators.
Assignment operators table
Operator keywords.
Three of the compound assignment operators have keyword equivalents. They are:
C++ specifies these operator keywords as alternative spellings for the compound assignment operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, the alternative spellings are keywords; use of <iso646.h> or the C++ equivalent <ciso646> is deprecated. In Microsoft C++, the /permissive- or /Za compiler option is required to enable the alternative spelling.
Simple assignment
The simple assignment operator ( = ) causes the value of the second operand to be stored in the object specified by the first operand. If both objects are of arithmetic types, the right operand is converted to the type of the left, before storing the value.
Objects of const and volatile types can be assigned to l-values of types that are only volatile , or that aren't const or volatile .
Assignment to objects of class type ( struct , union , and class types) is performed by a function named operator= . The default behavior of this operator function is to perform a member-wise copy assignment of the object's non-static data members and direct base classes; however, this behavior can be modified using overloaded operators. For more information, see Operator overloading . Class types can also have copy assignment and move assignment operators. For more information, see Copy constructors and copy assignment operators and Move constructors and move assignment operators .
An object of any unambiguously derived class from a given base class can be assigned to an object of the base class. The reverse isn't true because there's an implicit conversion from derived class to base class, but not from base class to derived class. For example:
Assignments to reference types behave as if the assignment were being made to the object to which the reference points.
For class-type objects, assignment is different from initialization. To illustrate how different assignment and initialization can be, consider the code
The preceding code shows an initializer; it calls the constructor for UserType2 that takes an argument of type UserType1 . Given the code
the assignment statement
can have one of the following effects:
Call the function operator= for UserType2 , provided operator= is provided with a UserType1 argument.
Call the explicit conversion function UserType1::operator UserType2 , if such a function exists.
Call a constructor UserType2::UserType2 , provided such a constructor exists, that takes a UserType1 argument and copies the result.

Compound assignment
The compound assignment operators are shown in the Assignment operators table . These operators have the form e1 op = e2 , where e1 is a non- const modifiable l-value and e2 is:
an arithmetic type
a pointer, if op is + or -
a type for which there exists a matching operator *op*= overload for the type of e1
The built-in e1 op = e2 form behaves as e1 = e1 op e2 , but e1 is evaluated only once.
Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type, or it must be a constant expression that evaluates to 0. When the left operand is of an integral type, the right operand must not be of a pointer type.
Result of built-in assignment operators
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value. These operators have right-to-left associativity. The left operand must be a modifiable l-value.
In ANSI C, the result of an assignment expression isn't an l-value. That means the legal C++ expression (a += b) += c isn't allowed in C.
Expressions with binary operators C++ built-in operators, precedence, and associativity C assignment operators
Was this page helpful?
Additional resources
study guides for every class
That actually explain what's on your next test, compound assignment operators, from class:, ap computer science a.
Compound assignment operators are shorthand notations that combine an arithmetic operation with the assignment operator. They allow you to perform an operation and assign the result to a variable in a single step.
congrats on reading the definition of Compound Assignment Operators . now let's actually learn it.
Related terms
This operator adds the value on the right side of it to the variable on the left side, and then assigns the result back to that variable.
-= operator : This operator subtracts the value on the right side of it from the variable on the left side, and then assigns the result back to that variable.
This operator multiplies the value on the right side of it with the variable on the left side, and then assigns the result back to that variable.
" Compound Assignment Operators " also found in:
Practice questions ( 1 ).
- Which arithmetic operators do not have compound assignment operators?
© 2024 Fiveable Inc. All rights reserved.
Ap® and sat® are trademarks registered by the college board, which is not affiliated with, and does not endorse this website..
Compound assignment operators
The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue.
The following table shows the operand types of compound assignment expressions:
Note that the expression
is equivalent to
The following table lists the compound assignment operators and shows an expression using each operator:
Although the equivalent expression column shows the left operands (from the example column) twice, it is in effect evaluated only once.
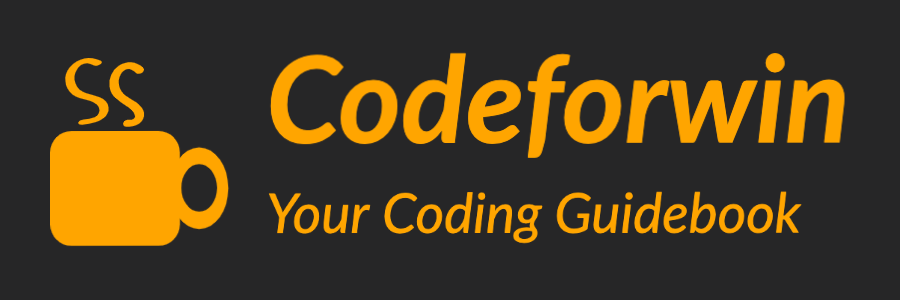
Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.

IMAGES
COMMENTS
Jul 15, 2018 · Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Aug 2, 2021 · The result of a compound-assignment operation has the value and type of the left operand. #define MASK 0xff00 n &= MASK; In this example, a bitwise-inclusive-AND operation is performed on n and MASK, and the result is assigned to n. The manifest constant MASK is defined with a #define preprocessor directive. See also. C Assignment Operators
Sep 23, 2017 · Output: 20 Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type-casting is automatically done by compile.
Augmented assignment (or compound assignment) is the name given to certain assignment operators in certain programming languages (especially those derived from C).An augmented assignment is generally used to replace a statement where an operator takes a variable as one of its arguments and then assigns the result back to the same variable.
Mar 1, 2024 · Compound assignment. The compound assignment operators are shown in the Assignment operators table. These operators have the form e1 op= e2, where e1 is a non-const modifiable l-value and e2 is: an arithmetic type. a pointer, if op is + or -a type for which there exists a matching operator *op*= overload for the type of e1
Definition. Compound assignment operators are shorthand notations that combine an arithmetic operation with the assignment operator. They allow you to perform an operation and assign the result to a variable in a single step.
In modern C, or even moderately ancient C, += is a compound assignment operator, and =+ is parsed as two separate tokens. = and +. Punctuation tokens are allowed to be adjacent. So if you write: x += y; it's equivalent to. x = x + y; except that x is only evaluated once (which can matter if it's a more complicated expression). If you write: x =+ y;
Compound assignment operators. The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue. The following table shows the operand types of ...
Aug 12, 2017 · Shorthand assignment operator. C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. int a = 5; a = a + 2;
The compound operators are different in two ways, which we see by looking more precisely at their definition. The Java language specification says that: The compound assignment E1 op= E2 is equivalent to [i.e. is syntactic sugar for] E1 = (T) ((E1) op (E2)) where T is the type of E1, except that E1 is evaluated only once.